To "AI RAG" a website URL, you need to use a process called Retrieval Augmented Generation (RAG) where you essentially extract relevant content from the website using web scraping, then store that content in a vector database, allowing an AI model to retrieve and reference the information when responding to related queries; this typically involves using libraries like BeautifulSoup in Python to parse the HTML structure and extract text, followed by processing the text into embeddings for efficient retrieval within the vector store.
The RAG Recipe: Key Ingredients and Steps
Web Scraping: Gathering the Website Ingredients
Text Processing & Embeddings: Preparing the Ingredients
Vector Store: Organizing the Ingredients for Easy Access
Querying & Response Generation: Cooking Up Answers
Let's dive into each step:
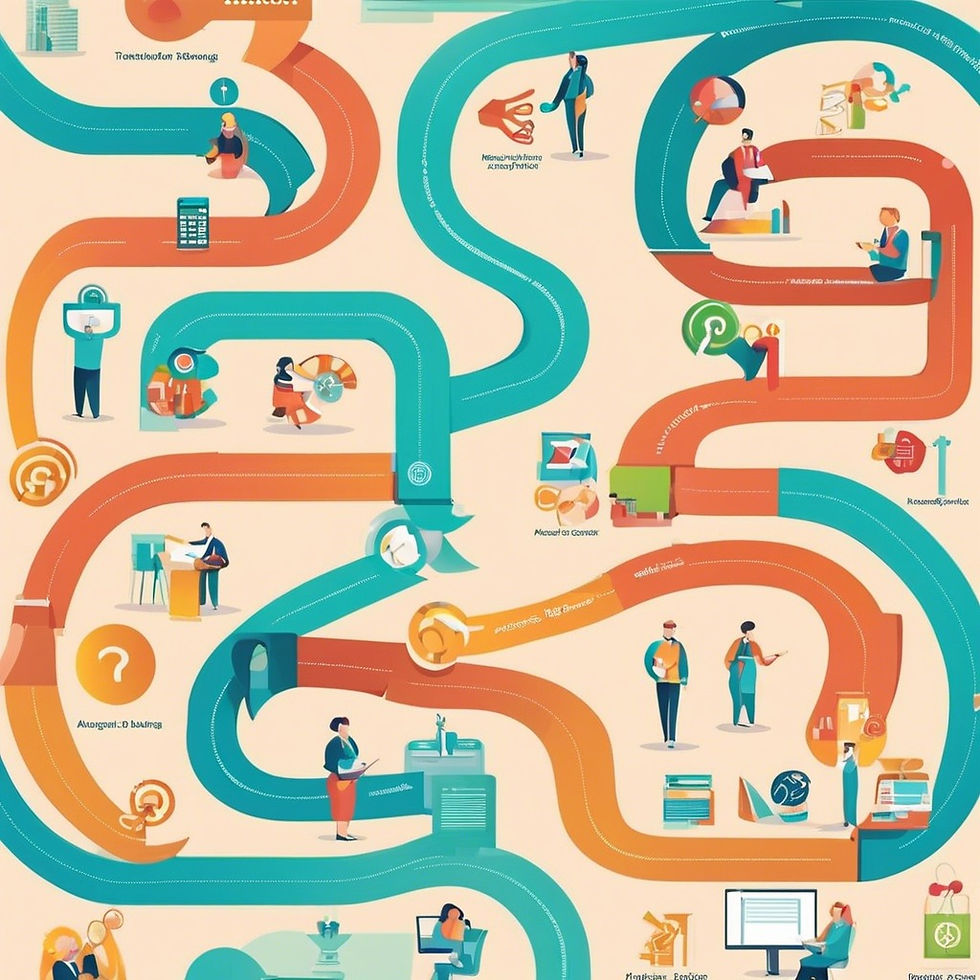
Key steps involved:
Web Scraping:
Access the URL: Use a library like requests to fetch the HTML content of the website at the given URL.
Parse HTML: Use a parser like BeautifulSoup to navigate through the HTML structure and extract the desired text content (like paragraphs, headings, etc.).
Clean Data: Remove unnecessary elements like tags, whitespace, and irrelevant information to prepare the text for processing.
Open Source Tools:
requests (Python Library): This is your web browser in code! It fetches the website's HTML code.
BeautifulSoup4 (Python Library): This tool helps you parse (understand) the HTML structure, making it easy to pick out the text you want.
How-to Steps:
Access the URL: Use requests to get the website's HTML content. import requests
from bs4 import BeautifulSoup
url = "YOUR_WEBSITE_URL_HERE" # Replace with the website you want to RAG
response = requests.get(url)
html_content = response.text
Parse HTML: Use BeautifulSoup4 to navigate the HTML and extract text.
soup = BeautifulSoup(html_content, 'html.parser')
# Example: Extract all paragraph text
paragraphs = soup.find_all('p')
website_text = ' '.join([p.text for p in paragraphs])
# You can customize this to target specific parts of the website
# (e.g., headings, articles, etc.) by inspecting the HTML structure.
Clean Data: Websites have extra HTML tags and messy formatting. Clean it up! You can use Python's string functions or libraries like re (regular expressions).
import re
# Remove extra whitespace, newlines, and tags (basic cleaning)
cleaned_text = re.sub(r'\s+', ' ', website_text).strip()
Text Processing and Embeddings:
Chunking: Large blocks of text are hard for AI to process efficiently. We break the text into smaller, meaningful chunks (like paragraphs or sentences).
Text Embeddings: AI understands numbers, not words. Embeddings convert each text chunk into a list of numbers (a vector) that represents the text's meaning. Similar text chunks will have similar vectors.
Vector Store:
Storage: Store these text vectors in a vector database like Faiss, Chroma, or Milvus, which allows efficient similarity searches based on the embedding vectors.
Imagine a library where books are organized not by title or author, but by meaning. That's what a vector store is! It's a special database designed to store and quickly search through vectors (our text embeddings).
Open Source Tools:
Faiss (Library by Facebook AI): Fast and efficient for similarity search in large vector datasets.
ChromaDB (Python Library): Easy-to-use vector database, great for getting started.
Milvus (Open-source Vector Database): Scalable and feature-rich vector database.
import chromadb
# Initialize ChromaDB in-memory (for simplicity)
client = chromadb.Client()
collection = client.create_collection("website_content")
# Add embeddings and corresponding text chunks
collection.add(embeddings=embeddings.tolist(), documents=text_chunks, ids=[str(i) for i in range(len(text_chunks))])
Querying and Response Generation:
Query Embedding: When a user asks a question related to the website content, embed their query into a vector using the same model.
Retrieval: Search the vector store to find the most relevant text chunks based on the query's embedding.
Augmentation: Append the retrieved text chunks to the user's query and provide this combined context to a large language model (LLM) like GPT-3.
Response Generation: The LLM generates a response using the combined context, drawing information directly from the website content.
Important Considerations:
Website Structure: Every website is built differently. You might need to adjust your scraping code to target the specific content you need. Inspect the website's HTML (right-click on a webpage and select "Inspect" or "View Page Source" in your browser) to understand its structure.
API Availability: Some websites offer APIs (Application Programming Interfaces) to access their data in a structured way. If available, using an API is usually more reliable and efficient than scraping.
Ethical Scraping: Be respectful! Check a website's robots.txt file (e.g., www.example.com/robots.txt) to see if they disallow scraping. Don't overload websites with too many requests.
RAG Frameworks: For more complex RAG applications, explore frameworks like LangChain or LlamaIndex. These frameworks simplify the process and offer more advanced features.
RAG: Unlock Website Knowledge with AI
RAG is a powerful technique to make AI models more knowledgeable and context-aware. By combining web scraping, vector databases, and language models, you can create intelligent search and question-answering systems that tap into the vast information available online, all while using open-source tools! Start experimenting and see what you can build!
Comments